Objective-C - if...else 语句
if 语句后可以跟一个可选的else 语句,它在布尔表达式为假时执行。
语法
Objective-C 编程语言中 if...else 语句的语法是 −
if(boolean_expression) { /* statement(s) will execute if the boolean expression is true */ } else { /* statement(s) will execute if the boolean expression is false */ }
如果布尔表达式的计算结果为true,则将执行if 块代码,否则将执行else 块代码 .
Objective-C 编程语言将任何非零 和非空 值假定为true,并且如果它是 零 或null,则假定为false 值。
流程图
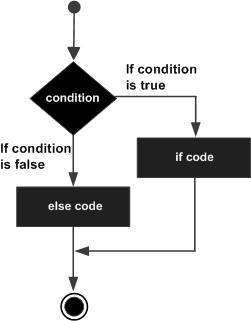
示例
#import <Foundation/Foundation.h> int main () { /* local variable definition */ int a = 100; /* check the boolean condition */ if( a < 20 ) { /* if condition is true then print the following */ NSLog(@"a is less than 20\n" ); } else { /* if condition is false then print the following */ NSLog(@"a is not less than 20\n" ); } NSLog(@"value of a is : %d\n", a); return 0; }
当上面的代码被编译和执行时,会产生如下结果 −
2013-09-07 22:04:10.199 demo[3537] a is not less than 20 2013-09-07 22:04:10.200 demo[3537] value of a is : 100
if...else if...else 语句
if 语句后面可以跟一个可选的else if...else 语句,这对于使用单个 if...else if 测试各种条件非常有用 陈述。
当使用 if , else if , else 语句时,有几点需要牢记 −
if 可以有 0 个或 1 个,它必须在任何其他 if 之后。
一个 if 可以有零到多个其他 if,并且它们必须在 else 之前。
一旦 else if 成功,剩下的 else if 或 else 都不会被测试。
语法
在 Objective-C 编程语言中 if...else if...else 语句的语法是 −
if(boolean_expression 1) { /* Executes when the boolean expression 1 is true */ } else if( boolean_expression 2) { /* Executes when the boolean expression 2 is true */ } else if( boolean_expression 3) { /* Executes when the boolean expression 3 is true */ } else { /* executes when the none of the above condition is true */ }
示例
#import <Foundation/Foundation.h> int main () { /* local variable definition */ int a = 100; /* check the boolean condition */ if( a == 10 ) { /* if condition is true then print the following */ NSLog(@"Value of a is 10\n" ); } else if( a == 20 ) { /* if else if condition is true */ NSLog(@"Value of a is 20\n" ); } else if( a == 30 ) { /* if else if condition is true */ NSLog(@"Value of a is 30\n" ); } else { /* if none of the conditions is true */ NSLog(@"None of the values is matching\n" ); } NSLog(@"Exact value of a is: %d\n", a ); return 0; }
当上面的代码被编译和执行时,会产生如下结果 −
2013-09-07 22:05:34.168 demo[8465] None of the values is matching 2013-09-07 22:05:34.168 demo[8465] Exact value of a is: 100
objective_c_decision_making.html