Pygame - 绘制形状
使用pygame.draw模块中的函数,可以在游戏窗口中绘制矩形、圆形、椭圆形、多边形、直线等不同形状 −
绘制一个矩形 | rect(surface, color, rect) |
绘制一个多边形 | polygon(surface, color, points) |
绘制一个圆 | circle(surface, color, center, radius) |
绘制一个椭圆 | ellipse(surface, color, rect) |
绘制一个椭圆弧 | arc(surface, color, rect, start_angle, stop_angle) |
绘制一条直线 | line(surface, color, start_pos, end_pos, width) |
示例
下面的例子使用这些函数来绘制不同的形状 −
import pygame pygame.init() screen = pygame.display.set_mode((400, 300)) done = False red = (255,0,0) green = (0,255,0) blue = (0,0,255) white = (255,255,255) while not done: for event in pygame.event.get(): if event.type == pygame.QUIT: done = True pygame.draw.rect(screen, red, pygame.Rect(100, 30, 60, 60)) pygame.draw.polygon(screen, blue, ((25,75),(76,125),(275,200),(350,25),(60,280))) pygame.draw.circle(screen, white, (180,180), 60) pygame.draw.line(screen, red, (10,200), (300,10), 4) pygame.draw.ellipse(screen, green, (250, 200, 130, 80)) pygame.display.update()
输出
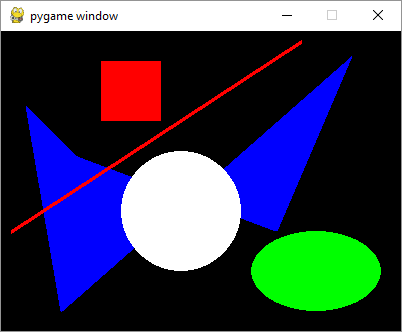
如果将可选的整数参数添加到函数中,则将使用指定的颜色作为轮廓颜色绘制形状。 数字对应于形状内部的轮廓粗细和背景颜色。
pygame.draw.rect(screen, red, pygame.Rect(100, 30, 60, 60),1) pygame.draw.circle(screen, white, (180,180), 60,2) pygame.draw.ellipse(screen, green, (250, 200, 130, 80),5)
输出
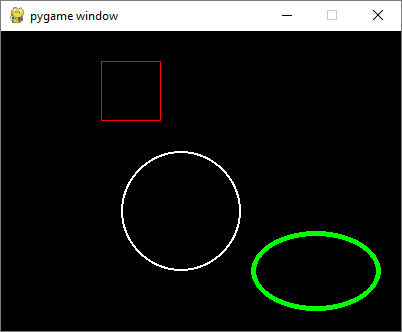